Fundamentals of Embedded Software with the ARM Cortex-M3, 1st edition
Published by Pearson (February 2, 2012) © 2013
- Daniel W. Lewis
eTextbook
- Easy-to-use search and navigation
- Add notes and highlights
- Search by keyword or page
- Hardcover, paperback or looseleaf edition
- Affordable rental option for select titles
- Free shipping on looseleafs and traditional textbooks
For sophomore-level courses in Assembly Language Programming in Computer Science, Embedded Systems Design, Real-Time Analysis, Computer Engineering, or Electrical Engineering curricula. Requires prior knowledge of C, C++, or Java. Allows instructors to easily introduce embedded systems into an already packed curriculum, and provides a way to cover the procedural style still necessary in upper-division courses.
This book is intended to provide a highly motivating context in which to learn procedural programming languages. The ultimate goal of this text is to lay a foundation that supports the multi-threaded style of programming and high-reliability requirements of embedded software. It presents assembly the way it is most commonly used in practice - to implement small, fast, or special-purpose routines called from a main program written in a high-level language such as C. Students not only learn that assembly still has an important role to play, but their discovery of multi-threaded programming, preemptive and non-preemptive systems, shared resources, and scheduling helps sustain their interest, feeds their curiosity, and strengthens their preparation for subsequent courses on operating systems, real-time systems, networking, and microprocessor-based design.
- An alternative to a more traditional course on assembly language programming. This text is intended to serve as the basis for a sophomore level course in a computer science, computer engineering, or electrical engineering curriculum. This course is envisioned as a replacement for the traditional course on computer organization and assembly language programming.
- Presents assembly the way it is most commonly used in practice - to implement small, fast, or special-purpose routines called from a main program written in a high-level language such as C. This approach affords time within both the text and the course to cover assembly in the context of embedded software. As a result, students not only learn that assembly still has an important role to play, but their discovery of multi-threaded programming, preemptive and non-preemptive systems, shared resources, and scheduling helps sustain their interest, feeds their curiosity, and strengthens their preparation for subsequent courses on operating systems, real-time systems, networking, and microprocessor-based design.
- Allows instructors to easily introduce embedded systems into an already packed curriculum, and provides a way to cover the procedural style still necessary in some upper-division courses. At most institutions the popular approach is to now use an object-oriented programming language such as C++ or Java. Despite the change, it is not uncommon to find such languages still in use in industry. At the author's institution, the traditional assembly language course was redesigned around the material in this book; it not only created room in an already packed curriculum to cover the procedural approach and to introduce the popular topic of embedded systems, but it has also offered an opportunity to strengthen student comprehension of parameter passing, scope, and memory allocation schemes that they were first introduced to in CS1 and CS2.
- Emphasizes those features of C that are employed more frequently in embedded applications, and introduces the procedural style through examples and programming assignments that include large amounts of pre-written source code.The text assumes that students already know how to program in C, C++, or Java, and that the similarity among the low-level syntax of those languages makes it relatively easy to move to C from either C++ or Java.
- Programming Assignments and the Companion Web Site. The text is complemented by a collection of programming assignments described in the appendices. Most of the source code for each assignment is provided on the Web Site. Some assignments address related topics not covered in the text, such as A/D conversion, feedback control, and processor utilization. The content can be accessed through the book’s companion web site at: www.pearsonhighered.com/lewis
Virtually a completely rewritten edition, featuring the following significant changes:
- Covers the architecture and instruction set of the ARM Cortex-M3 v7 processor, replacing the earlier coverage of the Intel IA32 processor. The 32-bit ARM processor was selected because (1) 75% of the embedded systems designed between 2004 and 2010 used 32-bit processors, (2) the use of ARM processors is growing rapidly, from 19% of all embedded applications in 2007 to more than 35% in 2010, and (3) the ARM® Cortex-M3TM is specifically designed for real-time embedded applications.
- Covers features that make the ARM Cortex-M3 processor well-suited for embedded applications, including conditional execution that avoids flushing the instruction pipeline, interrupt “tail-chaining”, “late arrival processing” of interrupts, and “bit-banding” for addressing individual bits in memory and I/O.
- Covers the ARM Procedure Call Standard and the appropriate choice of registers for function parameters, return values, and temporary variables.
- Adds a new chapter on “Implementing Arithmetic”, and reorganizes and expands the coverage of assembly language programming and I/O from three to four chapters.
- Expanded coverage of binary addition and subtraction, and new material on using shifts, adds and subtracts as alternatives to multiplication by a constant, reciprocal multiplication as alternative to division by a constant, and a fully worked example about multiplication of fixed-point real numbers.
- Updated to reflect C99 standards for the C programming language.
- Vastly expanded set of problems at the end of chapters 1-12, including answers to selected problems.
- The Companion Website includes 14 new laboratory programming assignments based on Texas Instruments’ $49 EKI-LM3S811 evaluation kit that includes the LM3S811 evaluation board, the source code of TI’s Stellaris Peripheral Driver Library, and the Kickstart edition of IAR Systems’ Embedded Workbench Integrated Development Environment. The LM3S811 evaluation board provides programmable timers, a thumbwheel-driven potentiometer, A/D converters, a graphic display, user and reset push-buttons, a user LED, parallel ports, serial UARTs, a USB port and a JTAG connector.
- Uses the open source FreeRTOS real-time kernel to develop multi-threaded laboratory assignments that investigate scheduling, processor utilization, thread starvation, unbounded priority inversion, deadlock, mutexes, semaphores, and queues.
- Complete plans are provided on the Companion Website for an easy to assemble and inexpensive breadboard that is used in the last four laboratory assignments to develop multi-threaded applications for feedback control of a small DC motor.
1.1 WHAT IS AN EMBEDDED SYSTEM?
1.2 WHAT’S UNIQUE ABOUT THE DESIGN GOALS FOR EMBEDDED SOFTWARE?
1.3 What Does "Real-Time" Mean?
1.4 What Does "multithreading" mean?
1.5 HOW POWERFUL ARE EMBEDDED PROCESSORS?
1.6 WHAT PROGRAMMING LANGUAGES ARE USED?
1.7 HOW IS BUILDING AN EMBEDDED APPLICATION DIFFERENT?
1.8 HOW BIG ARE TYPICAL EMBEDDED PROGRAMS?
PROBLEMS
2 Data Representation
2.1 FIXED-PRECISION BINARY NUMBERS2.2 POSITIONAL NUMBER SYSTEMS
2.2.1 Binary-to-Decimal Conversion
2.2.2 Decimal-to-Binary Conversion
2.2.3 Hexadecimal — A Shorthand for Binary
2.2.4 Fixed Precision, Rollover and Overflow
2.3 BINARY REPRESENTATION OF INTEGERS
2.3.1 Signed Integers
2.3.2 Positive and Negative Representations of the Same Magnitude
2.3.3 Interpreting the Value of a 2’s-Complement Number
2.3.4 Changing the Sign of Numbers with Integer and Fractional Parts
2.3.5 Binary Addition and Subtraction
2.3.6 Range and Overflow
2.4 BINARY REPRESENTATION OF REAL NUMBERS
2.4.1 Floating-Point Real Numbers
2.4.2 Fixed-Point Real Numbers
2.5 ASCII REPRESENTATION OF TEXT
2.6 BINARY-CODED DECIMAL (BCD)
PROBLEMS
3 Implementing Arithmetic
3.1 2’s Complement and hardware complexity3.2 MULTIPLICATION AND DIVISION
3.2.1 Signed vs. Unsigned Multiplication
3.2.2 Shifting Instead of Multiplying or Dividing by Powers of 2
3.2.3 Multiplying by an Arbitrary Constant
3.2.4 Dividing by an Arbitrary Constant
3.3 ARITHMETIC FOR FIXED-POINT REALS
3.3.1 Fixed-Point Using a Universal 16.16 Format
3.3.2 Fixed-Point Using a Universal 32.32 Format
3.3.3 Multiplication of 32.32 Fixed Point Reals
3.3.4 Example: Multiplying two 4.4 Fixed Point Reals
PROBLEMS
4 Getting the Most Out of C
4.1 Integer Data Types
4.1.1 Integer Range and the Standard Header File LIMITS.H
4.2 BOOLEAN Data Types
4.3 Mixing Data Types
4.4 Manipulating Bits in Memory
4.4.1 Testing Bits
4.4.2 Setting, Clearing, and Inverting Bits
4.4.3 Extracting Bits
4.4.4 Inserting Bits
4.5 Manipulating Bits in INPUT/OUTPUT PORTS
4.5.1 Write-Only I/O Devices
4.5.2 I/O Devices Differentiated by Reads Versus Writes
4.5.3 I/O Devices Differentiated by Sequential Access
4.5.4 I/O Devices Differentiated by Bits in the Written Data
4.6 Accessing Memory-Mapped I/O Devices
4.6.1 Accessing Data Using a Pointer
4.6.2 Arrays, Pointers, and the “Address of” Operator
4.7 Structures
4.7.1 Packed Structures
4.7.2 Bit Fields
4.8 Variant Access
4.8.1 Casting the Address of an Object
4.8.2 Using Unions
Problems
5 Programming in Assembly
Part 1: Computer Organization
5.1 Memory
5.1.1 Data Alignment
5.2 The Central Processing Unit (CPU)
5.2.1 Other Registers
5.2.2 The Fetch-Execute Cycle
5.3 Input/Output (I/O)
5.4 Introduction to the ARM® CortexTM- M3 V7M Architecture
5.4.1 Internal Organization
5.4.2 Instruction Pipelining
5.4.3 Memory Model
5.4.4 Bit-Banding
5.5 ARM ASSEMBLY LANGUAGE
5.5.1 Instruction Formats and Operands
5.5.2 Translating Assembly into Binary
Problems
6 Programming in Assembly
Part 2: Data Manipulation
6.1 LOADING CONSTANTS INTO REGISTERS
6.2 LOADING MEMORY DATA INTO REGISTERS
6.3 STORING DATA FROM REGISTERS TO MEMORY
6.4 CONVERTING SIMPLE C ASSIGNMENT STATEMENTS INTO ARM ASSEMBLY
6.5 MEMORY ADDRESS CALCULATIONS
6.6 MEMORY ADDRESSING EXAMPLES
6.6.1 Translating C Pointer Expressions to Assembly
6.6.2 Translating C Subscript Expressions to Assembly
6.6.3 Translating Structure References to Assembly
6.7 STACK INSTRUCTIONS
6.8 DATA PROCESSING INSTRUCTIONS
6.8.1 Updating the Flags in the APSR
6.8.2 Arithmetic Instructions
6.8.3 Bit Manipulation Instructions
6.8.4 Shift Instructions
6.8.5 Bitfield Manipulation Instructions
6.8.6 Miscellaneous Bit, Byte and Halfword Instructions
PROBLEMS
7 Programming in Assembly
Part 3: Control Structures
7.1 INSTRUCTION SEQUENCING
7.2 IMPLEMENTING DECISIONS
7.2.1 Conditional Branch Instructions
7.2.2 If-Then and If-Then-Else Statements
7.2.3 Compound Conditionals
7.1.4 The “If-Then” (IT) Instruction
7.2 IMPLEMENTING LOOPS
7.2.1 Speeding Up Array Access
7.3 IMPLEMENTING FUNCTIONS
7.3.1 Function Call and Return
7.3.2 Register Usage
7.3.3 Parameter Passing
7.3.4 Return Values
7.3.5 Temporary Variables
7.3.6 Preserving Registers
PROBLEMS
8 Programming in Assembly
Part 4: I/O Programming
8.1 THE CORTEX-M3 I/O HARDWARE
8.1.1 Interrupts and Exceptions
8.1.2 Thread and Handler Modes
8.1.3 Entering the Exception Handler
8.1.4 Returning from the Exception Handler
8.1.5 Latency Reduction
8.1.6 Priorities and Nested Exceptions
8.2 SYNCHRONIZATION, TRANSFER RATE, AND LATENCY
8.3 BUFFERS AND QUEUES
8.3.1 Double Buffering
8.4 ESTIMATING I/O PERFORMANCE CAPABILITY
8.4.1 Polled Waiting Loops
8.4.2 Interrupt-Driven I/O
8.4.3 Direct Memory Access
8.4.4 Comparison of Methods
PROBLEMS
9 Concurrent Software
9.1 FOREGROUND/BACKGROUND SYSTEMS
9.1.1 Thread State and Serialization
9.1.2 Managing Latency
9.1.3 Interrupt Overrun
9.1.4 Moving Work into the Background
9.2 MULTI-THREADED PROGRAMMING
9.2.1 Concurrent Execution of Independent Threads
9.2.2 Context Switching
9.2.3 Non-preemptive (Cooperative) Multithreading
9.2.4 Preemptive Multithreading
9.3 SHARED RESOURCES AND CRITICAL SECTIONS
9.3.1 Disabling Interrupts
9.3.2 Disabling Task Switching
9.3.3 Spin Locks
9.3.4 Mutex Objects
9.3.5 Semaphores
PROBLEMS
10 Scheduling
10.1 THREAD STATES
10.2 PENDING THREADS
10.3 CONTEXT SWITCHING
10.4 ROUND-ROBIN SCHEDULING
10.5 PRIORITY-BASED SCHEDULING
10.5.1 Resource Starvation
10.5.2 Priority Inversion
10.5.3 The Priority Ceiling Protocol
10.5.4 The Priority Inheritance Protocol
10.6 ASSIGNING PRIORITIES
10.6.1 Deadline-Driven Scheduling
10.6.2 Rate-Monotonic Scheduling
10.7 DEADLOCK
10.8 WATCHDOG TIMERS
PROBLEMS
11 Memory Management
11.1 OBJECTS IN C
11.2 SCOPE
11.2.1 Refining Local Scope
11.2.2 Refining Global Scope
11.3 LIFETIME
11.4 AUTOMATIC ALLOCATION
11.4.1 Storage Class “Register”
11.5 STATIC ALLOCATION
11.6 THREE PROGRAMS TO DISTINGUISH STATIC FROM AUTOMATIC
11.6.1 Object Creation
11.6.2 Object Initialization
11.6.3 Object Destruction
11.7 DYNAMIC ALLOCATION
11.7.1 Fragmentation
11.7.2 Memory Allocation Pools
11.8 AUTOMATIC ALLOCATION WITH VARIABLE SIZE (alloca)
11.8.1 Variable-Size Arrays
11.9 RECURSIVE FUNCTIONS AND MEMORY ALLOCATION
PROBLEMS
12 Shared Memory
12.1 RECOGNIZING SHARED OBJECTS
12.2 REENTRANT FUNCTIONS
12.3 READ-ONLY DATA
12.3.1 Type Qualifier "const"
12.4 CODING PRACTICES TO AVOID
12.4.1 Functions That Keep Internal State in Local Static Objects
12.4.2 Functions That Return the Address of a Local Static Object
12.5 ACCESSING SHARED MEMORY
12.5.1 The Effect of Processor Architecture
12.5.2 Read-Only and Write-Only Access
12.5.3 Type Qualifier “volatile”
PROBLEMS
13 System Initialization
13.1 MEMORY LAYOUT
13.2 THE CPU AND VECTOR TABLE
13.3 C RUN-TIME ENVIRONMENT
13.3.1 Copying Initial Values from Non-Volatile Memory into the Data Region
13.3.2 Zeroing Uninitialized Statics
13.3.3 Setting Up a Heap
13.4 SYSTEM TIMER
13.5 OTHER PERIPHERAL DEVICES
Prior to joining the University in 1975, Lewis worked for six years at General Electric’s Aerospace Division where he designed a fault-tolerant clocking system for one of the first triple-redundant automatic landing systems for commercial aircraft. He has consulted for a number of Bay Area companies, including the Singer-Link Company, where his design of new algorithms and a corresponding modular array of VLSI circuits became the basis of a new product line of real-time computer graphics systems.
Need help? Get in touch
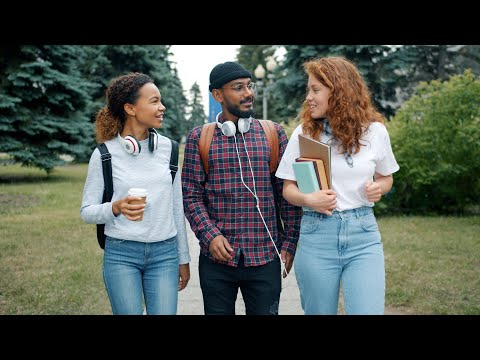
Pearson eTextbook: What’s on the inside just might surprise you
They say you can’t judge a book by its cover. It’s the same with your students. Meet each one right where they are with an engaging, interactive, personalized learning experience that goes beyond the textbook to fit any schedule, any budget, and any lifestyle.
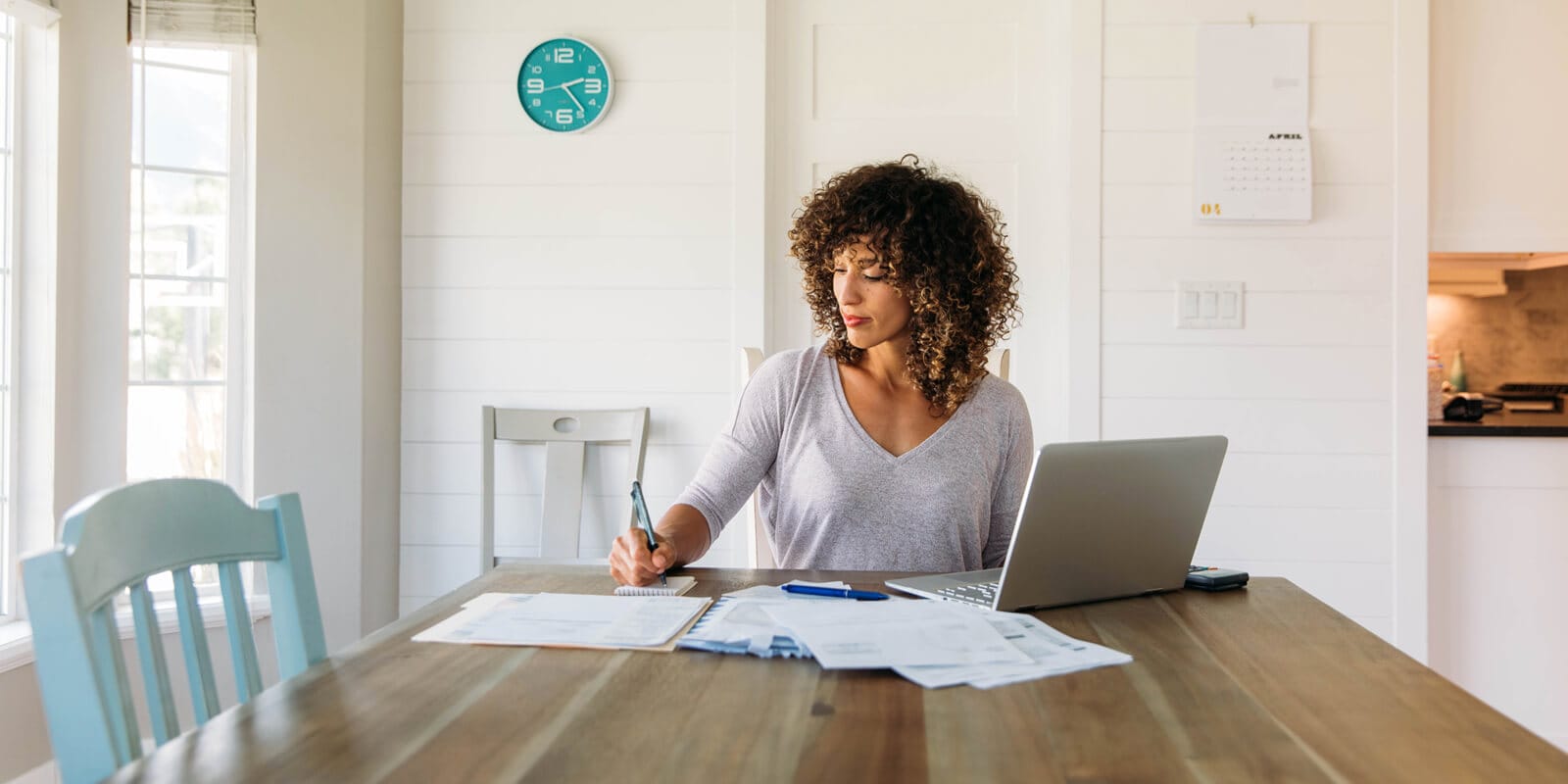
Digital Learning NOW
Extend your professional development and meet your students where they are with free weekly Digital Learning NOW webinars. Attend live, watch on-demand, or listen at your leisure to expand your teaching strategies. Earn digital professional development badges for attending a live session.