Switch content of the page by the Role togglethe content would be changed according to the role
Effective Python: 90 Specific Ways to Write Better Python, 2nd edition
Published by Addison-Wesley Professional (November 15, 2019) © 2020
- Brett Slatkin
eTextbook
$47.99
- Available for purchase from all major ebook resellers, including InformIT.com.
- To request a review copy, click on the "Request a Review Copy" button.
$55.99
- A print text (hardcover or paperback)
- Free shipping
- Also available for purchase as an ebook from all major ebook resellers, including InformIT.com
Updated and Expanded for Python 3
This second edition of Effective Python will help you master a truly “Pythonic” approach to programming, harnessing Python’s full power to write exceptionally robust and well-performing code. Using the concise, scenario-driven style pioneered in Scott Meyers’ best-selling Effective C++, Brett Slatkin brings together 90 Python best practices, tips, and shortcuts, and explains them with realistic code examples so that you can embrace Python with confidence.
Drawing on years of experience building Python infrastructure at Google, Slatkin uncovers little-known quirks and idioms that powerfully impact code behavior and performance. You’ll understand the best way to accomplish key tasks so you can write code that’s easier to understand, maintain, and improve. In addition to even more advice, this new edition substantially revises all items from the first edition to reflect how best practices have evolved.
Key features include
- 30 new actionable guidelines for all major areas of Python
- Detailed explanations and examples of statements, expressions, and built-in types
- Best practices for writing functions that clarify intention, promote reuse, and avoid bugs
- Better techniques and idioms for using comprehensions and generator functions
- Coverage of how to accurately express behaviors with classes and interfaces
- Guidance on how to avoid pitfalls with metaclasses and dynamic attributes
- More efficient and clear approaches to concurrency and parallelism
- Solutions for optimizing and hardening to maximize performance and quality
- Techniques and built-in modules that aid in debugging and testing
- Tools and best practices for collaborative development
Effective Python will prepare growing programmers to make a big impact using Python.
- Python best practices, patterns, shortcuts, and "pythonic" idioms you won't find anywhere else
- Covers Python algorithms, objects, concurrency, collaboration, built-in modules, and much more
- Guides you to a far deeper understanding of the Python language, so you know why its unique idioms and rules of thumb make sense
- Follows the enormously popular "Effective" format proven in Scott Meyers' classic Effective C++
Why write a second edition?
1.There have been a large number of new language features added to Python. At the time the first edition was written (2014), Python 3.4 was the latest version of Python. When this second edition is ready to be published, the latest version will be version 3.7. Python 3.5 and 3.6 added a large number of significant language changes, specifically the async and await keywords, which fundamentally changed the nature of the language. Effective Python will begin to feel old as these language features increase in popularity.
2.The former version of Python, version 2, is being deprecated permanently in April 2020. This means that everyone who’s still using Python 2 needs to finish porting their code over to Python 3. See “Standard changes” section below for full details. This deprecation was expected when the first edition was written, but that was only at the half-way point in the expected lifetime of Python 2.7 (which was first released in 2010). This second edition will be published almost at the very end of Python 2’s life, meaning we can relegate Python 2 information to an appendix.
3.Python has continued to increase in popularity. It was the #8 ranked language in the TIOBE index in 2014, and it’s now the #5 most popular. It was the #4 ranked language in the RedMonk index in 2014, and now it’s the #3 most popular. Python continues to be used by major products and companies, with some recent examples being Instagram and TensorFlow (an incredibly popular machine learning library from Google). The PyCon conference is also more popular than ever.
4.The importance of supporting Windows users has increased. The first edition of the book and sample code focused on programmers who have UNIX-like environments, such as Linux or Mac OS X, which were by far the most popular environments used by Python programmers. However, as Python has grown in its appeal over the past few years, especially internationally, programmers are using Python on Windows more than ever. The Python core developers have also added many language improvements to make using Python on Windows feel more natural. Thus, the second edition should have sample code that works equally well in the three major environments.
Changes to the Python Standard
The Python programming language is currently undergoing a move from version 2 of the language to version 3, a slow process that has been underway for the past 10+ years. Python 3 fixes a bunch of important problems present in Python 2, causing the versions to be slightly incompatible. Critically, Python 2 will be considered deprecated and abandoned by the community on April 12, 2020. When Effective Python was first publised (March 2015), a large number of the most popular packages used by the Python community had made the move to Python 3, but there were still many popular ones that were lagging behind (notably Django and NumPy). As of this writing (August 2017), all but a tiny number of popular modules have ported to Python 3. This makes me feel confident that the deprecation will go forward as planned.
Since Effective Python was published, the Python language has continued to evolve, with major new features added in each point release (e.g., 3.1, 3.2, 3.3). The language just had version 3.6 released in December 2016, and version 3.7 is scheduled to be released in June 2018. Python releases are scheduled to be roughly 18 months apart. That means version 3.8 likely won’t be released until 2020.
By the time the second edition of this book will be ready to be published (estimated early 2019), Python 3.7 will have been out for 6 months, and Python 2 will have one year left of life. Python version 2 will likely still be popular, since people will still have another year to port their code over to the new version.
The first edition of this book chose to cover both version 2 and version 3 of Python. Python 2.7 (the final point version of Python 2) had originally been released in 2010 and was still very popular in 2015. For the second edition of this book, I believe I can remove all of the Python-2 specific content from the primary book and put it into a special appendix that serves as a historical reference. This is also where I can put advice on how to port Python 2 code over to Python 3 and adopt the best practices that I recommend.
Preface
Chapter 1 Pythonic Thinking
Chapter 2 Lists and Dictionaries
Chapter 3 Functions
Chapter 4 Comprehensions and Generators
Chapter 5 Classes and Interfaces
Chapter 6 Metaclasses and Attributes
Chapter 7 Concurrency and Parallelism
Chapter 8 Robustness and Performance
Chapter 9 Testing and Debugging
Chapter 10 Collaboration
Index
Chapter 1 Pythonic Thinking
Chapter 2 Lists and Dictionaries
Chapter 3 Functions
Chapter 4 Comprehensions and Generators
Chapter 5 Classes and Interfaces
Chapter 6 Metaclasses and Attributes
Chapter 7 Concurrency and Parallelism
Chapter 8 Robustness and Performance
Chapter 9 Testing and Debugging
Chapter 10 Collaboration
Index
Brett Slatkin is a principal software engineer at Google. He is the technical co-founder of Google Surveys, the co-creator of the PubSubHubbub protocol, and he launched Google’s first cloud computing product (App Engine). Fourteen years ago, he cut his teeth using Python to manage Google’s enormous fleet of servers. Outside of his day job, he likes to play piano and surf (both poorly). He also enjoys writing about programming-related topics on his personal website (https://onebigfluke.com). He earned his B.S. in computer engineering from Columbia University in the City of New York. He lives in San Francisco.
Need help? Get in touch
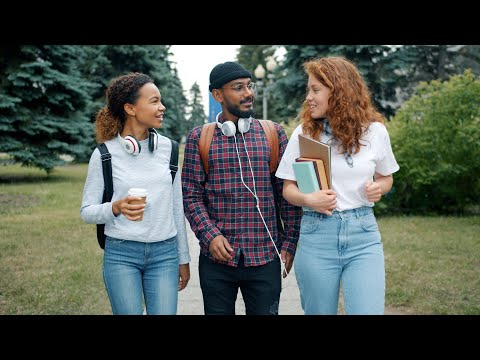
Privacy and cookies
By watching, you agree Pearson can share your viewership data for marketing and analytics for one year, revocable by deleting your cookies.
Pearson eTextbook: What’s on the inside just might surprise you
They say you can’t judge a book by its cover. It’s the same with your students. Meet each one right where they are with an engaging, interactive, personalized learning experience that goes beyond the textbook to fit any schedule, any budget, and any lifestyle.
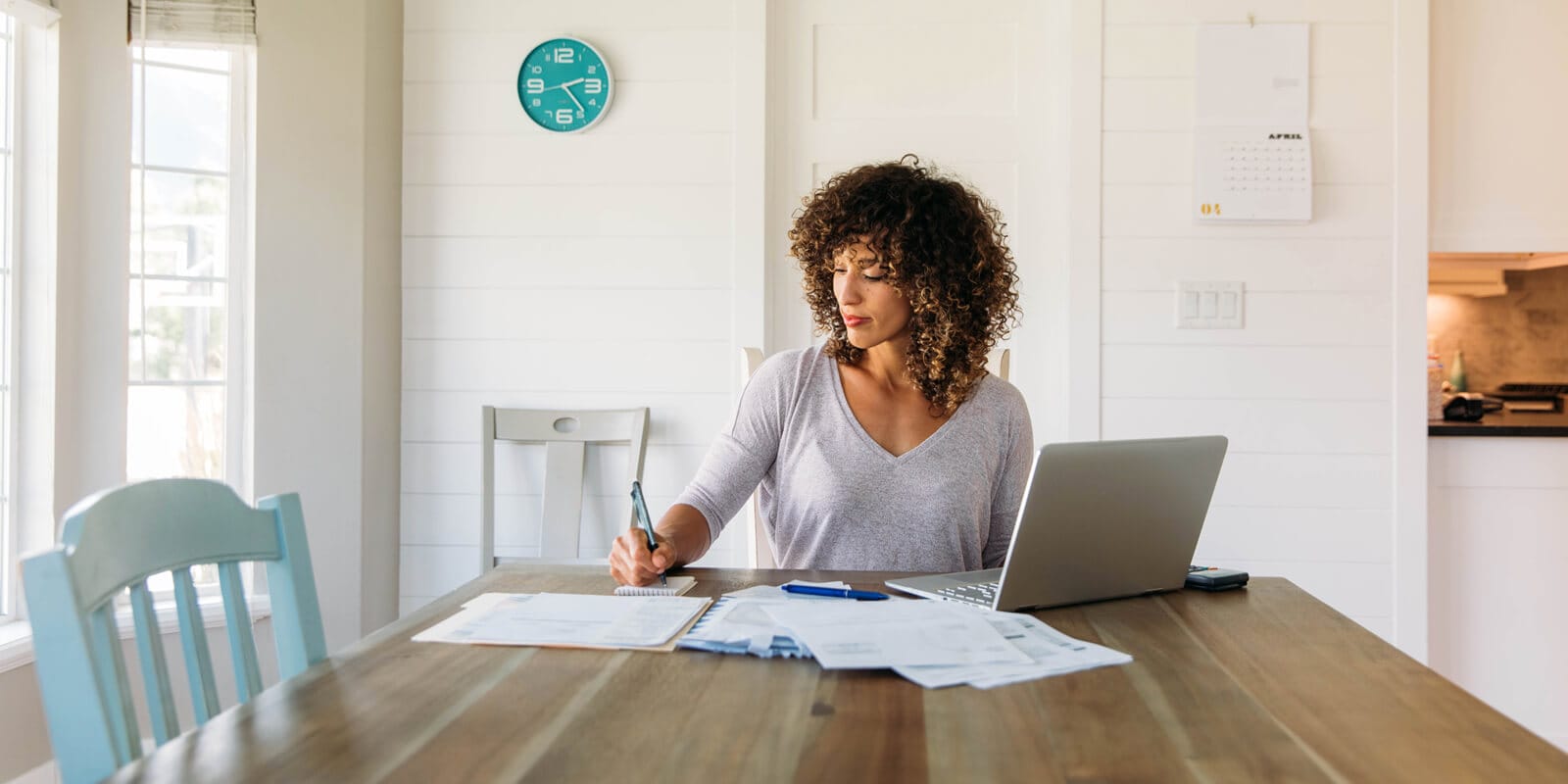
Digital Learning NOW
Extend your professional development and meet your students where they are with free weekly Digital Learning NOW webinars. Attend live, watch on-demand, or listen at your leisure to expand your teaching strategies. Earn digital professional development badges for attending a live session.