Switch content of the page by the Role togglethe content would be changed according to the role
Data Structures and Other Objects Using C++, 4th edition
Published by Pearson (February 24, 2010) © 2011
- Michael Main
$170.66
- Hardcover, paperback or looseleaf edition
- Affordable rental option for select titles
- Free shipping on looseleafs and traditional textbooks
Need help? Get in touch
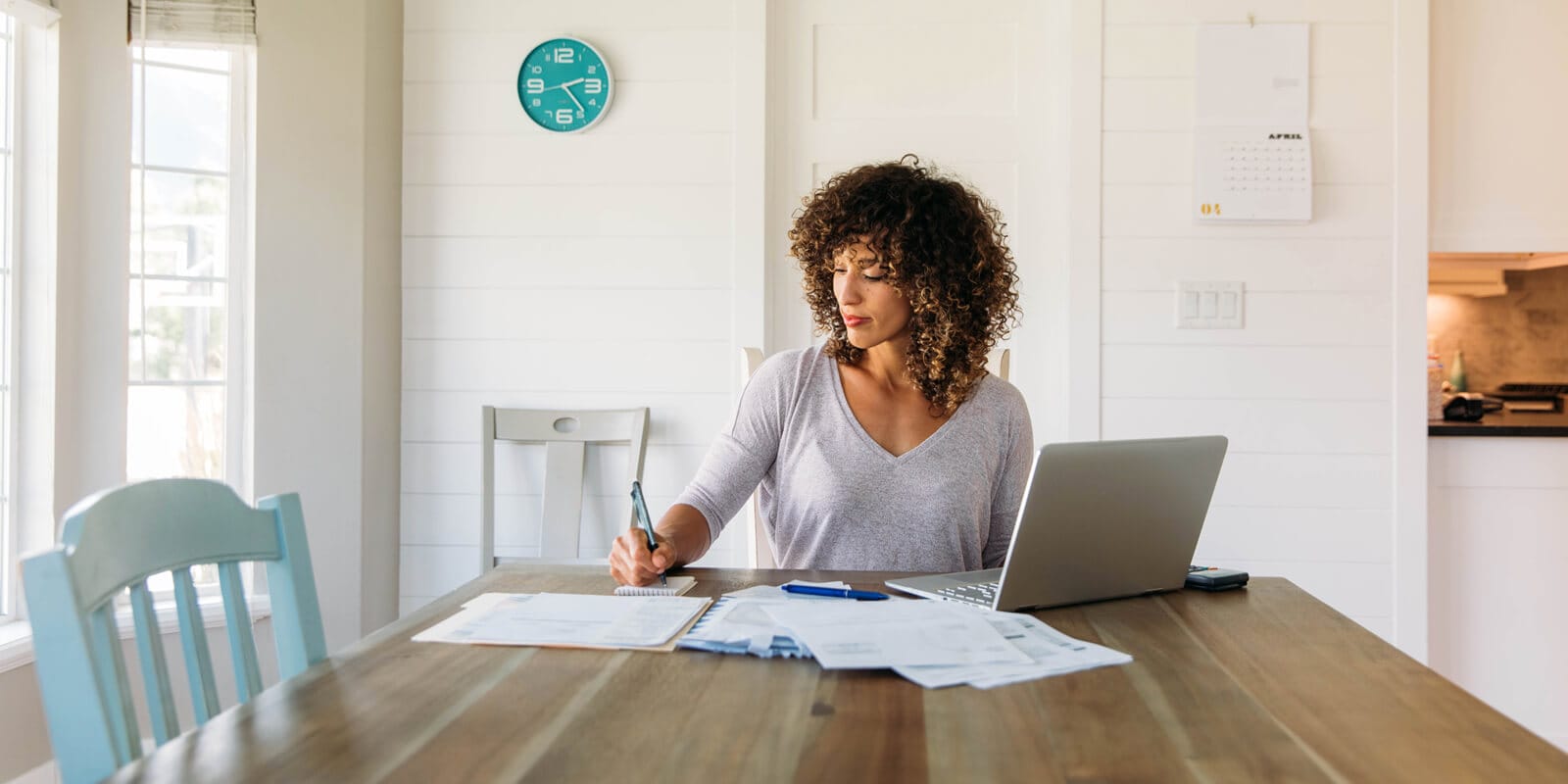
Digital Learning NOW
Extend your professional development and meet your students where they are with free weekly Digital Learning NOW webinars. Attend live, watch on-demand, or listen at your leisure to expand your teaching strategies. Earn digital professional development badges for attending a live session.