Data Structures with C++ Using STL, 2nd edition
Published by Pearson (July 17, 2001) © 2002
- William H. Ford
- Hardcover, paperback or looseleaf edition
- Affordable rental option for select titles
- Free shipping on looseleafs and traditional textbooks
For CS2/Data Structures courses using C++.
This book uses a modern object-oriented approach to data structures, unified around the notion of the Standard Template Library (STL) container classes. The book presents a systematic development of data structures supported by numerous examples and complete programs. The authors separate the applications of a data structure from its implementation. In the later chapters, the book transitions students into the study of applied algorithms. This creates a bridge to subsequent courses in advanced data structures and algorithms.
- NEW - Data structures presented using the model of the Standard Template Library (STL)—Over the course of the book, student master the use and implementation of the STL container classes.
-
Teaches students the modern approach to data structures. Ex.___
-
- NEW - Simple algorithms integrated throughout the text.
-
Includes an applied study of interesting and classical algorithms that illustrate the data structures using only simple mathematical concepts. (Big-O notation is introduced intuitively). Ex.___
-
- NEW - Many additional figures integrated into the presentation.
-
Lead students through the concepts. Ex.___
-
- NEW - ADT (Abstract Data Type) for each data structure—Immediately used to solve appropriate problems.
-
Book provides students with many examples before presenting the corresponding implementation so that students see a data structure unfold and are then able to trace back through the examples when looking at the implementation. Ex.___
-
- NEW - Early and accessible introduction to templates and iterators.
-
Allows students to understand and use powerful abstractions available in modern data structures. Ex.___
-
- NEW - Later chapters (Chapters 11 - 17) supplement the CS2 course.
-
Appropriate material for an advanced data structures and algorithms course. Ex.___
-
- Use of modern C++ constructs in developing data structures and their applications—Provides enough language detail to sufficiently understand the constructs. A fuller explanation of language details available on the Companion Website.
-
Reflects the type of data structures that students would use in the real world. Ex.___
-
- Extensive pedagogy—Includes many complete programs with output, case studies, review exercises with solutions, extensive written exercises and programming exercises, programming projects, and glossary of key terms.
-
Helps students learn, review, and retain material. Ex.___
-
- Data structures presented using the model of the Standard Template Library (STL)—Over the course of the book, student master the use and implementation of the STL container classes.
-
Teaches students the modern approach to data structures. Ex.___
-
- Simple algorithms integrated throughout the text.
-
Includes an applied study of interesting and classical algorithms that illustrate the data structures using only simple mathematical concepts. (Big-O notation is introduced intuitively). Ex.___
-
- Many additional figures integrated into the presentation.
-
Lead students through the concepts. Ex.___
-
- ADT (Abstract Data Type) for each data structure—Immediately used to solve appropriate problems.
-
Book provides students with many examples before presenting the corresponding implementation so that students see a data structure unfold and are then able to trace back through the examples when looking at the implementation. Ex.___
-
- Early and accessible introduction to templates and iterators.
-
Allows students to understand and use powerful abstractions available in modern data structures. Ex.___
-
- Later chapters (Chapters 11 - 17) supplement the CS2 course.
-
Appropriate material for an advanced data structures and algorithms course. Ex.___
-
Preface.
1. Introduction to Data Structures.
2. Object Design Techniques.
3. Introduction to Algorithms.
4. The Vector Container.
5. Pointers and Dynamic Memory.
6. The List Container and Iterators.
7. Stacks.
8. Queues and Priority Queues.
9. Linked Lists.
10.Binary Trees.
11. Associative Containers.
12. Advanced Associative Structures.
13. Inheritance and Abstract Classes.
14. Heaps Binary Files and Bit Sets.
15. Recursive Algorithms.
16. Graphs.
Index.
Professor William Ford and Professor William Topp are faculty members with the Computer Science Department, University of the Pacific, Stockton, California. They have also written Introduction to Computing with C++ and Object Technology (Prentice Hall, 1999) and Assembly Language and Systems Programming for the M68000 Family (Jones and Bartlett, 1992).
Need help? Get in touch
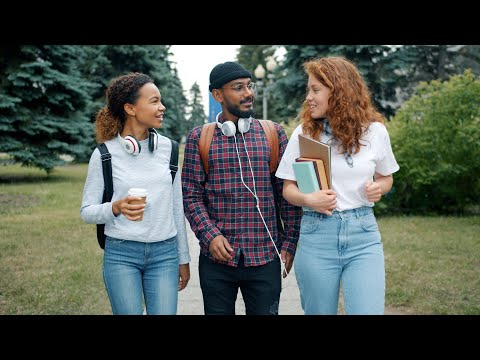
Pearson eTextbook: What’s on the inside just might surprise you
They say you can’t judge a book by its cover. It’s the same with your students. Meet each one right where they are with an engaging, interactive, personalized learning experience that goes beyond the textbook to fit any schedule, any budget, and any lifestyle.
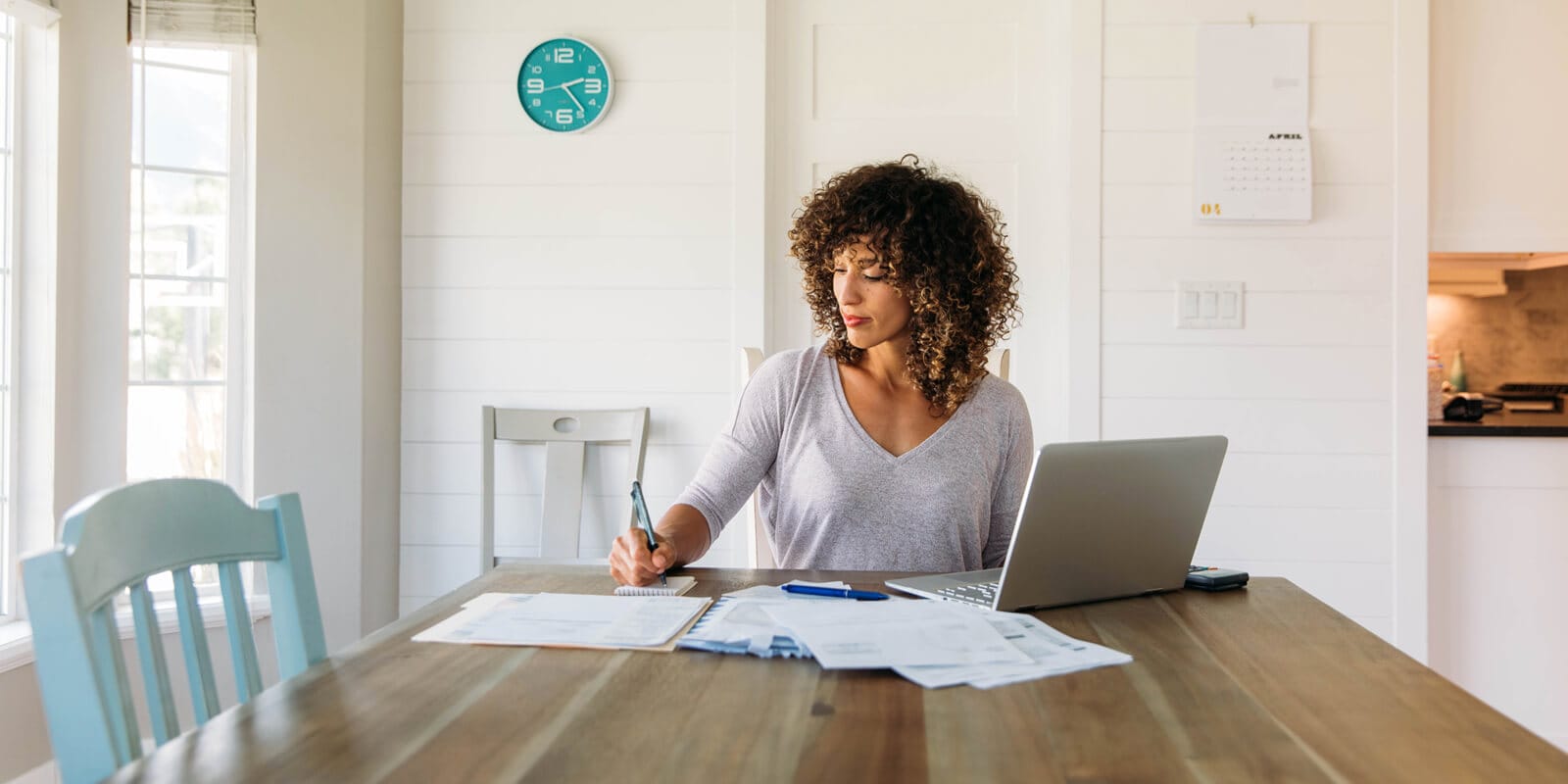
Digital Learning NOW
Extend your professional development and meet your students where they are with free weekly Digital Learning NOW webinars. Attend live, watch on-demand, or listen at your leisure to expand your teaching strategies. Earn digital professional development badges for attending a live session.