Starting Out with C++ from Control Structures to Objects, 9th edition
Published by Pearson (February 13, 2017) © 2018
- Tony Gaddis Haywood Community College
- Hardcover, paperback or looseleaf edition
- Affordable rental option for select titles
About the Book
Teach C++ from the fundamentals to the details
- Written with clear, easy-to-understand language, and rich with example programs that are concise, practical, and real-world oriented.
- The hundreds of Example Programs in the text are designed to highlight the topic currently being studied. Source code for these programs is provided so that students can run the programs themselves.
- Students not only learn how to implement the features and constructs of C++, but why and when to use them.
- Teaches C++ in a step-by-step fashion. Each chapter covers a major set of topics and builds knowledge as the student progresses through the book but still allows for flexibility past Chapter 7.
Features for student success
- UPDATED! The material on the Standard Template Library (STL) has been completely rewritten and expanded into its own chapter.
- Concept Statements, Checkpoints, Notes, and Warnings throughout the book all call out important pieces of information for the student
- Program Output is a sample of its screen output shown after each example program. This immediately shows the student how the program should function.
- In the Spotlight sections provides a programming problem and a detailed, step by step analysis showing the student how to solve it.
- VideoNotes developed specifically for this book are available at www.pearsonhighered.com/gaddis. Icons appear throughout the text alerting the student to videos about specific topics. Students can follow along with the author as he works through each tutorial in the videos.
- C++ Quick Reference Guide is printed on the last two pages of Appendix C in the book to provide a quick, easy reference to the C++ language.
- C++11 language features are introduced throughout the text with C++11 icons identifying the new features.
- Case studies that simulate real-world applications appear in many chapters throughout the text. These case studies are designed to highlight the major topics of the chapter in which they appear.
Give students opportunities to apply programming concepts and skills
- A thorough and diverse set of review questions, such as fill-in-the-blank and short answer, check the student’s mastery of the basic material presented in each chapter. These are followed by exercises requiring problem solving and analysis, such as the Algorithm Workbench, Predict the Output, and Find the Errors sections.
- EXPANDED! Several new programming problems have been added throughout the book
- Programming Challenges are designed to solidify the student’s knowledge of the topics currently being studied. Each chapter offers a pool of programming exercises. In most cases the assignments present real-world problems to be solved.
- Group Projects are intended to be constructed by a team of students. There are several group programming projects throughout the text and emulate the way many professional programs are written and encourages team work within the classroom.
- Software Development Project: Serendipity Booksellers is available for download from the book’s Companion Website at www.pearsonhighered.com/gaddis. This is an ongoing project that instructors can optionally assign to teams of students. It systematically develops a “real-world” software package: a point-of-sale program for the fictitious Serendipity Booksellers organization. When complete, the program will act as a cash register, manage an inventory database, and produce a variety of reports.
Also Available with MyLabProgramming
MyLab™ Programming is an online learning system designed to engage students and improve results. MyLabProgramming consists of programming exercises correlated to the concepts and objectives in this book. Through practice exercises and immediate, personalized feedback, MyLab Programming improves the programming competence of beginning students who often struggle with the basic concepts of programming languages.
- UPDATED! User Interface provides a new streamlined interface based on experienced user feedback. Course creation, configuration, and navigation are now easier than ever
- EXPANDED! Exercise Editor now allows you to easily create new programming exercises. In addition to assigning the hundreds of programming exercises already available in MyProgrammingLab, you can create and assign programming exercises to customize your course
- UPDATED! VideoNotes Tutorials provide step-by-step video tutorials specifically designed to enhance the programming concepts presented in Introduction to Java Programming. Students can view the entire problem-solving process outside of the classroom–when they need help the most
- Interactive Practice provides first-hand programming experience in an interactive online environment
- Immediate feedback for incorrect answers give students personalized feedback differentiating logical and compiler errors . The error messages include both the feedback from the compiler and plain English interpretations of likely causes for the incorrect answer
- NEW! The Plagiarism Detection Tool alerts instructors of potential plagiarism issues by checking:
- Students’ average submission rate
- Students’ average number of attempts until correct
- Pearson eText gives students access to their textbook anytime, anywhere. In addition to note taking, highlighting, and bookmarking, the Pearson eText offers interactive and sharing features. Rich media options let students watch lecture and example videos as they read or do their homework. Instructors can share their comments or highlights, and students can add their own, creating a tight community of learners in your class.
- The Pearson eText companion app allows existing subscribers to access their titles on an iPad or Android tablet for either online or offline viewing.
- Dynamic grading and assessment provide auto-grading of student assignments, saving you time and offering students immediate learning opportunities:
- A dynamic roster tracks their performance and maintains a record of submissions.
- The color-coded gradebook gives you a quick glance of your classes' progress. Easily drill down to receive information on a single student's performance or a specific problem. Gradebook results can be exported to Excel to use with your LMS.
Personalize learning with MyLab Programming.
MyLab™ Programming is an online learning system designed to engage students and improve results. MyLab Programming consists of programming exercises correlated to the concepts and objectives in this book. Through practice exercises and immediate, personalized feedback, MyLab Programming improves the programming competence of beginning students who often struggle with the basic concepts of programming languages.
- UPDATED! User Interface provides a new streamlined interface based on experienced user feedback. Course creation, configuration, and navigation are now easier than ever.
- EXPANDED! Exercise Editor now allows you to easily create new programming exercises. In addition to assigning the hundreds of programming exercises already available in MyProgrammingLab, you can create and assign programming exercises to customize your course.
- UPDATED! VideoNotes Tutorials provide step-by-step video tutorials specifically designed to enhance the programming concepts presented in Introduction to Java Programming. Students can view the entire problem-solving process outside of the classroom—when they need help the most.
- The Plagiarism Detection Tool alerts instructors of potential plagiarism issues by checking:
- Students’ average submission rate
- Students’ average number of attempts until correct
About the Book
- The material on the Standard Template Library (STL) has been completely rewritten and expanded into its own chapter. Previously, Chapter 16 covered exceptions, templates, and gave brief coverage to the STL. In this edition, Chapter 16 covers exceptions and templates, and Chapter 17 is a new chapter dedicated to the STL. The new chapter covers the following topics:
- The array and vector classes
- The various types of iterators
- Emplacement versus insertion
- The map , multimap , and unordered_map Classes
- The set , multiset , and unordered_set Classes
- Sorting and searching algorithms
- Permutation algorithms
- Set algorithms
- Using function pointers with STL algorithms
- Function objects, or functors
- Lambda expressions
- Chapter 2 now includes a discussion of alternative forms of variable initialization, including functional notation, and brace notation (also known as uniform initialization).
- Chapter 3 now mentions the round function, introduced in C++ 11.
- Chapter 7 now introduces array initialization much earlier.
- In Chapter 8, the bubble sort algorithm has been rewritten and improved.
- A new example of sorting and searching a vector of strings has been added to Chapter 8.
- In Chapter 9, the section on smart pointers now gives an overview of shared_ptr s and weak_ptr s, in addition to the existing coverage of unique_ptr s.
- In Chapter 10, a new In the Spotlight section on string tokenizing has been added.
- Chapter 10 now covers the string -to-number conversion functions that were introduced in C++ 11.
- The material on unions that previously appeared in Chapter 11 has been moved to Appendix K, available on the book’s companion Website.
- Chapter 13 has new sections covering:
- Member initialization lists.
- In-place initialization.
- Constructor delegation.
- Several new topics were added to Chapter 14, including:
- Rvalue references and move semantics.
- Checking for self-assignment when overloading the = operator.
- Using member initialization lists in aggregate classes.
- Chapter 15 includes a new section on constructor inheritance.
- Several new programming problems have been added throughout the book.
1. Introduction to Computers and Programming
2. Introduction to C++
3. Expressions and Interactivity
4. Making Decisions
5. Loops and Files
6. Functions
7. Arrays and Vectors
8. Searching and Sorting Arrays
9. Pointers
10. Characters, C-Strings, and More about the string Class
11. Structured Data
12. Advanced File Operations
13. Introduction to Classes
14. More about Classes
15. Inheritance, Polymorphism, and Virtual Functions
16. Exceptions and Templates
17. The Standard Template Library
18. Linked Lists
19. Stacks and Queues
20. Recursion
21. Binary Trees
Appendix A: The ASCII Character Set
Appendix B: Operator Precedence and Associativity
Quick References
Online The following appendices are available at www.pearsonhighered.com/gaddis.
Appendix C: Introduction to Flowcharting
Appendix D: Using UML in Class Design
Appendix E: Namespaces
Appendix F: Passing Command Line Arguments
Appendix G: Binary Numbers and Bitwise Operations
Appendix H: STL Algorithms
Appendix I: Multi-Source File Programs
Appendix J: Stream Member Functions for Formatting
Appendix K: Unions
Appendix L: Answers to Checkpoints
Appendix M: Answers to Odd Numbered Review Questions
Case Study 1: String Manipulation
Case Study 2: High Adventure Travel Agency–Part 1
Case Study 3: Loan Amortization
Case Study 4: Creating a String Class
Case Study 5: High Adventure Travel Agency–Part 2
Case Study 6: High Adventure Travel Agency–Part 3
Case Study 7: Intersection of Sets
Case Study 8: Sales Commission
Tony Gaddis is the principal author of the Starting Out With series of textbooks. Tony has nearly two decades of experience teaching computer science courses, primarily at Haywood Community College. He is a highly acclaimed instructor who was previously selected as the North Carolina Community College “Teacher of the Year” and has received the Teaching Excellence award from the National Institute for Staff and Organizational Development. The Starting Out With series includes introductory books covering C++, Java™, Microsoft® Visual Basic®, Microsoft® C#®, Python®, Programming Logic and Design, Alice, and App Inventor, all published by Pearson. More information about all these books can be found at www.pearsonhighered.com.
Need help? Get in touch
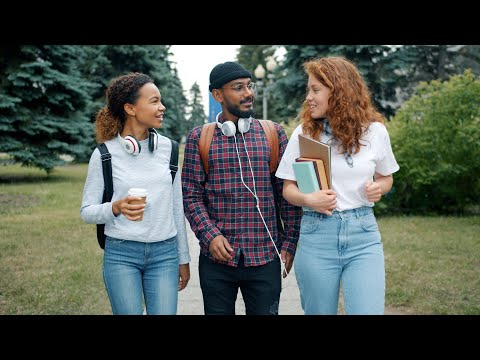
Pearson eTextbook: What’s on the inside just might surprise you
They say you can’t judge a book by its cover. It’s the same with your students. Meet each one right where they are with an engaging, interactive, personalized learning experience that goes beyond the textbook to fit any schedule, any budget, and any lifestyle.
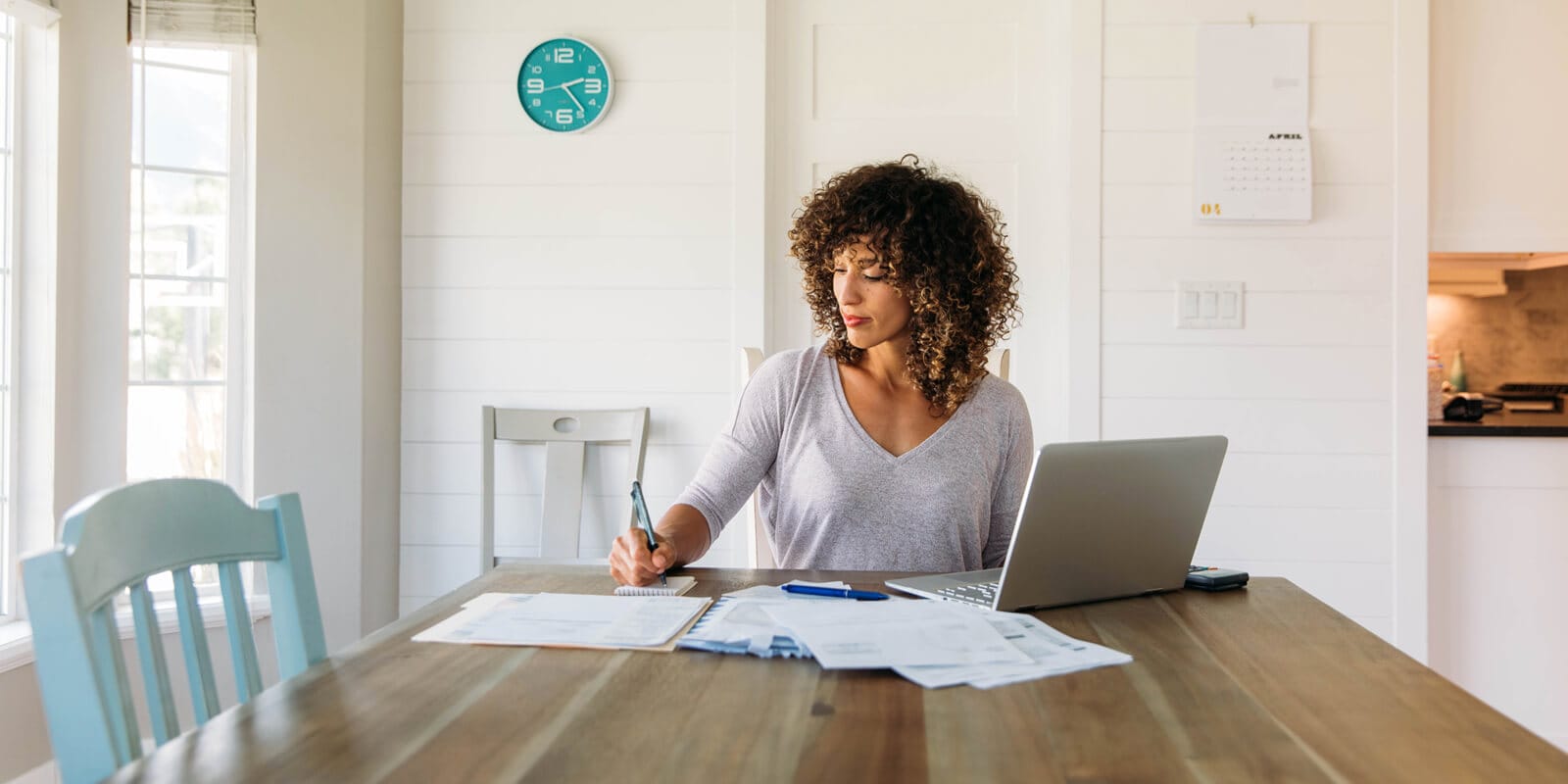
Digital Learning NOW
Extend your professional development and meet your students where they are with free weekly Digital Learning NOW webinars. Attend live, watch on-demand, or listen at your leisure to expand your teaching strategies. Earn digital professional development badges for attending a live session.