Programming in C, 4th edition
Published by Addison-Wesley Professional (August 18, 2014) © 2015
- Stephen G. Kochan
eTextbook
- Available for purchase from all major ebook resellers, including InformIT.com.
- To request a review copy, click on the "Request a Review Copy" button.
- A print text (hardcover or paperback)Â
- Free shipping
- Also available for purchase as an ebook from all major ebook resellers, including InformIT.com
Programming in C, Fourth Edition is a newly revised and updated edition of Steven Kochan's classic C programming tutorial — a book that has helped thousands of students master C over the past 25 years. This edition fully reflects the latest developments in the C programming and has been crafted to help students master C regardless of the platform they intend to use or the applications they intend to create—including mobile and gaming-device applications — where C's elegance and speed make it especially valuable. Kochan begins with the fundamentals, then covers every facet of C programming: variables, data types, arithmetic expressions, program looping, making decisions, arrays, functions, structures, character strings, pointers, operations on bits, the preprocessors, I/O, and more. Coverage also includes chapters on working with larger programs; debugging programs, and the fundamentals of object-oriented programming.
- The book that's helped programmers master C for 25+ years: now updated for even greater relevance and value
- Revamped examples and well-crafted exercises provide hands-on experience
- Begins with simple tasks and builds to pro-quality techniques
- Guide to common mistakes helps you build robust, reliable code without trial-and-error
- By Stephen Kochan, author of several best-selling programming tutorials, including Programming in Objective-C
-New material on C-based object-oriented programming languages (e.g., Objective-C)-Instructor materials Â
   Introduction 1
1 Some Fundamentals 5
   Programming 5
   Higher-Level Languages 5
   Operating Systems 6
   Compiling Programs 7
   Integrated Development Environments 10
   Language Interpreters 10
2 Compiling and Running Your First Program 11
   Compiling Your Program 12
   Running Your Program 12
   Understanding Your First Program 13
   Displaying the Values of Variables 15
   Comments 17
   Exercises 19
3 Variables, Data Types, and Arithmetic Expressions 21
   Understanding Data Types and Constants 21
       The Integer Type int 22
       The Floating Number Type float 23
       The Extended Precision Type double 23
       The Single Character Type char 24
       The Boolean Data Type _Bool 24
       Type Specifiers: long, long long, short, unsigned, and signed 26
   Working with Variables 29
   Working with Arithmetic Expressions 30
       Integer Arithmetic and the Unary Minus Operator 33
   Combining Operations with Assignment: The Assignment Operators 39
   Types _Complex and _Imaginary 40
   Exercises 40
4 Program Looping 43
   Triangular Numbers 43
   The for Statement 44
       Relational Operators 46
       Aligning Output 50
   Program Input 51
       Nested for Loops 53
       for Loop Variants 55
   The while Statement 56
   The do Statement 60
       The break Statement 62
       The continue Statement 62
   Exercises 63
5 Making Decisions 65
   The if Statement 65
       The if-else Construct 69
       Compound Relational Tests 72
       Nested if Statements 74
       The else if Construct 76
   The switch Statement 83
   Boolean Variables 86
   The Conditional Operator 90
   Exercises 92
6 Working with Arrays 95
   Defining an Array 96
       Using Array Elements as Counters 100
       Generating Fibonacci Numbers 103
       Using an Array to Generate Prime Numbers 104
   Initializing Arrays 106
   Character Arrays 108
       Base Conversion Using Arrays 109
       The const Qualifier 111
   Multidimensional Arrays 113
   Variable Length Arrays 115
   Exercises 117
7 Working with Functions 119
   Defining a Function 119
   Arguments and Local Variables 123
       Function Prototype Declaration 124
       Automatic Local Variables 124
   Returning Function Results 126
   Functions Calling Functions Calling... 130
       Declaring Return Types and Argument Types 133
       Checking Function Arguments 135
   Top-Down Programming 137
   Functions and Arrays 137
       Assignment Operators 141
       Sorting Arrays 143
       Multidimensional Arrays 146
   Global Variables 151
   Automatic and Static Variables 155
   Recursive Functions 158
   Exercises 161
8 Working with Structures 163
   The Basics of Structures 163
   A Structure for Storing the Date 164
       Using Structures in Expressions 166
   Functions and Structures 169
       A Structure for Storing the Time 175
   Initializing Structures 178
       Compound Literals 178
   Arrays of Structures 180
   Structures Containing Structures 183
   Structures Containing Arrays 185
   Structure Variants 189
   Exercises 190
9 Character Strings 193
   Revisiting the Basics of Strings 193
   Arrays of Characters 194
   Variable-Length Character Strings 197
       Initializing and Displaying Character Strings 199
       Testing Two Character Strings for Equality 202
       Inputting Character Strings 204
       Single-Character Input 206
       The Null String 211
   Escape Characters 215
   More on Constant Strings 217
   Character Strings, Structures, and Arrays 218
       A Better Search Method 221
   Character Operations 226
   Exercises 229
10 Pointers 233
   Pointers and Indirection 233
   Defining a Pointer Variable 234
   Using Pointers in Expressions 237
   Working with Pointers and Structures 239
       Structures Containing Pointers 241
       Linked Lists 243
   The Keyword const and Pointers 251
   Pointers and Functions 252
   Pointers and Arrays 258
       A Slight Digression About Program Optimization 262
       Is It an Array or Is It a Pointer? 262
       Pointers to Character Strings 264
       Constant Character Strings and Pointers 266
       The Increment and Decrement Operators Revisited 267
   Operations on Pointers 271
   Pointers to Functions 272
   Pointers and Memory Addresses 273
   Exercises 275
11 Operations on Bits 277
   The Basics of Bits 277
   Bit Operators 278
       The Bitwise AND Operator 279
       The Bitwise Inclusive-OR Operator 281
       The Bitwise Exclusive-OR Operator 282
       The Ones Complement Operator 283
       The Left Shift Operator 285
       The Right Shift Operator 286
       A Shift Function 286
       Rotating Bits 288
   Bit Fields 291
   Exercises 295
12 The Preprocessor 297
   The #define Statement 297
       Program Extendability 301
       Program Portability 302
       More Advanced Types of Definitions 304
       The # Operator 309
       The ## Operator 310
   The #include Statement 311
       System Include Files 313
   Conditional Compilation 314
       The #ifdef, #endif, #else, and #ifndef Statements 314
       The #if and #elif Preprocessor Statements 316
       The #undef Statement 317
   Exercises 318
13 Extending Data Types with the Enumerated Data Type, Type Definitions, and Data Type Conversions 319
   Enumerated Data Types 319
   The typedef Statement 323
   Data Type Conversions 325
       Sign Extension 327
       Argument Conversion 328
   Exercises 329
14 Working with Larger Programs 331
   Dividing Your Program into Multiple Files 331
       Compiling Multiple Source Files from the Command Line 332
   Communication Between Modules 334
       External Variables 334
       Static Versus Extern Variables and Functions 337
       Using Header Files Effectively 339
   Other Utilities for Working with Larger Programs 341
       The make Utility 341
       The cvs Utility 343
       Unix Utilities: ar, grep, sed, and so on 343
15 Input and Output Operations in C 345
   Character I/O: getchar() and putchar() 346
   Formatted I/O: printf() and scanf() 346
       The printf() Function 346
       The scanf() Function 353
   Input and Output Operations with Files 358
       Redirecting I/O to a File 358
       End of File 361
   Special Functions for Working with Files 362
       The fopen Function 362
   The getc() and putc() Functions 364
       The fclose() Function 365
       The feof Function 367
       The fprintf() and fscanf() Functions 367
       The fgets() and fputs() Functions 367
       stdin, stdout, and stderr 368
       The exit() Function 369
       Renaming and Removing Files 370
   Exercises 371
16 Miscellaneous and Advanced Features 373
   Miscellaneous Language Statements 373
       The goto Statement 373
       The null Statement 374
   Working with Unions 375
   The Comma Operator 378
   Type Qualifiers 379
       The register Qualifier 379
       The volatile Qualifier 379
       The restrict Qualifier 379
   Command-line Arguments 380
   Dynamic Memory Allocation 384
       The calloc() and malloc() Functions 385
       The sizeof Operator 385
       The free Function 387
   Exercises 389
17 Debugging Programs 391
   Debugging with the Preprocessor 391
   Debugging Programs with gdb 397
       Working with Variables 400
       Source File Display 401
       Controlling Program Execution 402
       Getting a Stack Trace 406
       Calling Functions and Setting Arrays and Structures 407
       Getting Help with gdb Commands 408
       Odds and Ends 410
18 Object-Oriented Programming 413
   What Is an Object Anyway? 413
   Instances and Methods 414
   Writing a C Program to Work with Fractions 416
   Defining an Objective-C Class to Work with Fractions 417
   Defining a C++ Class to Work with Fractions 421
   Defining a C# Class to Work with Fractions 424
A C Language Summary 427
B The Standard C Library 471
C Compiling Programs with gcc 495
D Common Programming Mistakes 499
E Resources 505
TOC, 9780321776419, 7/28/2014
Â
Stephen G. Kochan has been developing software with the C programming language for more than 30 years. He is the author of several best-selling titles on the C language, including Programming in C , Programming in Objective-C , and Topics in C Programming . He has also written extensively on Unix and is the author or coauthor of Exploring the Unix System and Unix Shell Programming .
Need help? Get in touch
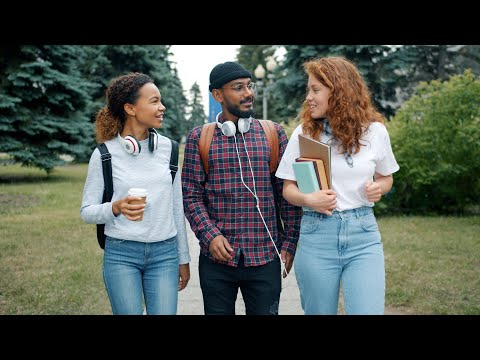
Pearson eTextbook: What’s on the inside just might surprise you
They say you can’t judge a book by its cover. It’s the same with your students. Meet each one right where they are with an engaging, interactive, personalized learning experience that goes beyond the textbook to fit any schedule, any budget, and any lifestyle.Â
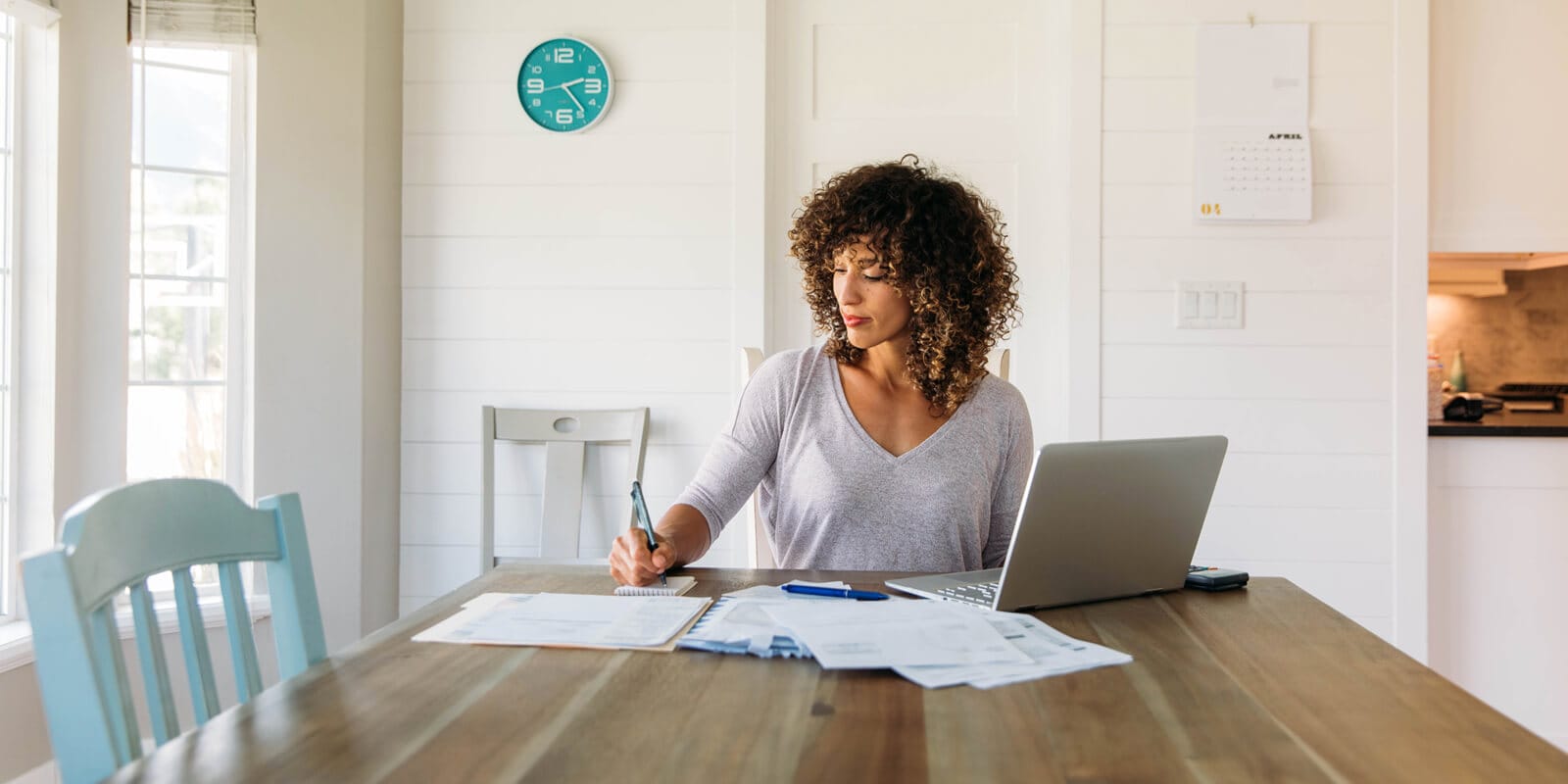
Digital Learning NOW
Extend your professional development and meet your students where they are with free weekly Digital Learning NOW webinars. Attend live, watch on-demand, or listen at your leisure to expand your teaching strategies. Earn digital professional development badges for attending a live session.